Well, the project has been finished for a few weeks now. You can download it at http://games.rit.edu/rocktropolis
The last few weeks of working on the project were crazy, but it turned out really great, and we got to show it off at GDC '07.
I might write up a little post mortem here when I get the chance, but for now... on to 3D!
Thursday, March 22, 2007
Friday, February 9, 2007
The latest
At this point, I have a file format defined that will control the flow of the game. It's based on the music, so you can define Background sounds that will play at a certain time and for a certain length, and then you can define Riffs which will transition to the battle mode and allow the player to play the notes. When the riff is over, it transitions back to the LEVEL state and play continues.
Things that need to be done (in no particular order):
1.Fix timing issue - there's a slight timing issue somewhere that's causing the music to become out of sync with my code after it loops a whole bunch of times. I have some ideas of what may be causing this, so hopefully I can track it down today. UPDATE: fixed on the dual core really fast machines in the lab, but it still gets out of sync on my laptop. I know a way to force a fix for this but I'm not going to implement it right now, maybe a bit later. Fixed, basically I handle looping on my own based on my own timer.
2.Riff Input for Multiple Key presses - so it plays more like guitar hero (ie, holding down the first note while hitting the second note doesn't cause you to miss a the second note) Also, riff input for chords. Feb 9th 2:15pm Done
3. Fix Menu transition timings - transitioning to the menu screws up timing in the battle mode at the moment, it's probably as simple as telling the riff and music to pause on outtro.
4. Note Display - the notes are just lines of color at the moment, which makes for difficult play if you forget the order of the colors. We're thinking of stacking them ala guitar hero. We need to figure out what we want the notes to actually look like, too. Also, we were talking about adding some cool effects to the notes, like some cool particles or something.
5. Character Animation State Machine - we need a nice state pattern for character animation, right now it's just kinda start and stop.
6.Score Class - we probably want a way to keep score, or something along those lines, so a nice singleton score class with methods like hitNote, missedNote, etc will probably work well. All Done! Includes guitar hero style multiplier and the ability to see how well the player performed on previous riffs, so we can tell if they learned them or not for the HUD.
7. LEVEL gameplay - we need some sort of gameplay in the LEVEL state. Chris and I discussed the possibility of a sidescroller shooter, where you have to shoot different colored notes at the enemies to kill them. This is probably going to be more work than we're expecting, so we should start on this asap. We're going to need things like an enemy class and bullet class, etc.
8. CONTENT - music, art. nuff said.
9. End Game? - what's going to happen when you finish the game? how's it going to end? we're not going to have enough time to implement a boss most likely, unless we throw a bunch of our already created features together to simulate a boss fight.
10. Menus
11. System Config checks - does your video card support our game? how much texture memory are we going to require, etc.
12. A way to get particle systems into the world without hard coding them - preferably a way that we can put them in the world using the world builder.
13. HUD
So, it's the end of week 8, we've got a week and a half left til milestone 3, and my other classes are starting to get pretty crazy. It's going to be an interesting next few weeks =)
Things that need to be done (in no particular order):
1.
2.
3. Fix Menu transition timings - transitioning to the menu screws up timing in the battle mode at the moment, it's probably as simple as telling the riff and music to pause on outtro.
4. Note Display - the notes are just lines of color at the moment, which makes for difficult play if you forget the order of the colors. We're thinking of stacking them ala guitar hero. We need to figure out what we want the notes to actually look like, too. Also, we were talking about adding some cool effects to the notes, like some cool particles or something.
5. Character Animation State Machine - we need a nice state pattern for character animation, right now it's just kinda start and stop.
6.
7. LEVEL gameplay - we need some sort of gameplay in the LEVEL state. Chris and I discussed the possibility of a sidescroller shooter, where you have to shoot different colored notes at the enemies to kill them. This is probably going to be more work than we're expecting, so we should start on this asap. We're going to need things like an enemy class and bullet class, etc.
8. CONTENT - music, art. nuff said.
9. End Game? - what's going to happen when you finish the game? how's it going to end? we're not going to have enough time to implement a boss most likely, unless we throw a bunch of our already created features together to simulate a boss fight.
10. Menus
11. System Config checks - does your video card support our game? how much texture memory are we going to require, etc.
12. A way to get particle systems into the world without hard coding them - preferably a way that we can put them in the world using the world builder.
13. HUD
So, it's the end of week 8, we've got a week and a half left til milestone 3, and my other classes are starting to get pretty crazy. It's going to be an interesting next few weeks =)
Thursday, January 25, 2007
State of things
Yesterday I added a Font Engine to our code that I wrote for MUPPETS this summer. It can create any font in any style using GDI, but renders it as sprites on the screen, which is fast.
I also added a Menu State and successful transitions between Game State and Menu State. We had a little issue with input and single key strokes, but Ada fixed that up quick and all works well.
I created a skeleton class for the Battle State, so next I'm going to start work on that. It's going to involve a lot of planning on the IO side, we need a file format for the notes that are going to be played (IE keys that need to be pressed), their timings, and any sound files that need to be played / faded, etc. So I'm going to start working on the basic pieces of this, and then hopefully this weekend we'll get some music going and go from there.
We're thinking of a more traditional side-scroller on-rails shooter type game for the "exploration" mode. So if someone else wants to start thinking about how that's going to work, and start thinking about collion detection for the bullets and stuff, that'd be cool. Some questions: How are we going to aim? (whammy bar?) How precise will the aiming be? Will it be timing based? What's going to happen when they play a note? if music is played, how is it going to sync? Will there be music in the background?
In other news, word on the street is that we may have lost our art guy, so that's going to be trouble.
But things are moving along. I don't have any word on the XNA port yet, but I'd rather keep working on the actual GAME part of things rather than rebuilding another engine. If there's no fun game, whats the point of 2 identical boring engines?
-p3000
I also added a Menu State and successful transitions between Game State and Menu State. We had a little issue with input and single key strokes, but Ada fixed that up quick and all works well.
I created a skeleton class for the Battle State, so next I'm going to start work on that. It's going to involve a lot of planning on the IO side, we need a file format for the notes that are going to be played (IE keys that need to be pressed), their timings, and any sound files that need to be played / faded, etc. So I'm going to start working on the basic pieces of this, and then hopefully this weekend we'll get some music going and go from there.
We're thinking of a more traditional side-scroller on-rails shooter type game for the "exploration" mode. So if someone else wants to start thinking about how that's going to work, and start thinking about collion detection for the bullets and stuff, that'd be cool. Some questions: How are we going to aim? (whammy bar?) How precise will the aiming be? Will it be timing based? What's going to happen when they play a note? if music is played, how is it going to sync? Will there be music in the background?
In other news, word on the street is that we may have lost our art guy, so that's going to be trouble.
But things are moving along. I don't have any word on the XNA port yet, but I'd rather keep working on the actual GAME part of things rather than rebuilding another engine. If there's no fun game, whats the point of 2 identical boring engines?
-p3000
Tuesday, January 23, 2007
State Switching Implemented
So, I spaced out in some class today to think about the State Switching implementation, and ran home to code it.
It's simple. Essentially there's a GameState Interface, which all States conform to. This interface contains methods like Draw, Update, OnReset, etc. Then we have a GameStateManager, which implements the GameState interface, and also contains a method for switching the current state. So when we want to switch from BATTLE mode to MENU mode, it's a simple method call, and the GameStateManager automatically forwards the calls appropriately.
It's all committed to SVN, so Ada, you can maybe help out Baker with the XNA port for now.
-p3000
It's simple. Essentially there's a GameState Interface, which all States conform to. This interface contains methods like Draw, Update, OnReset, etc. Then we have a GameStateManager, which implements the GameState interface, and also contains a method for switching the current state. So when we want to switch from BATTLE mode to MENU mode, it's a simple method call, and the GameStateManager automatically forwards the calls appropriately.
It's all committed to SVN, so Ada, you can maybe help out Baker with the XNA port for now.
-p3000
Monday, January 22, 2007
Sound Timing
I implemented a sound timing class tonight, and tested it out by creating a simple speaker looking object in GIMP and having it pulse at 120 beats per minute. I wrote a Speaker class that extends Sprite to take care of the graphical side of things. The sound timing class should be pretty robust and should help us with things from graphical pulsing to note timing detection.
I'm hoping this leads us in the right direction to start implementing gameplay. I asked Ada to work on a state pattern so we can have different states like the menu, rails mode, battle mode, etc. So maybe I'll start working on the battle mode stuff next now that I have a better idea of timing.
-p3000
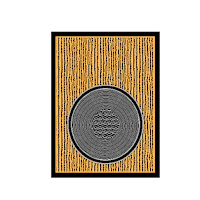
-p3000
Thursday, January 11, 2007
DirectInput Stuff Committed
I've finally committed my DirectInput implementation into svn. The controls are now F1 and F2 for moving, and 'h' for displaying the configuration UI. If you are running on your home machines you will be able to change and save configuration changes.
I will start making my own configuration UI just in case we can't get access to the required folder, and also looking at the input implementation for XNA.
Btw, I'm getting an error window pop-up when I minimize the game window and then restoring it. It's the same error that Ed had last on Wednesday night I think. Pete: don't know if you can fix it. On that note, I think we should have a topic on the forum that's on bugs that we found.
I will start making my own configuration UI just in case we can't get access to the required folder, and also looking at the input implementation for XNA.
Btw, I'm getting an error window pop-up when I minimize the game window and then restoring it. It's the same error that Ed had last on Wednesday night I think. Pete: don't know if you can fix it. On that note, I think we should have a topic on the forum that's on bugs that we found.
Wednesday, January 10, 2007
Player / Camera Path Design
I further thought through my position path issue, and I decided that a 3 point bezier curve would work best for the situation. The equation is simple, and we can get it to do exactly what we want. So now the question is implementation.
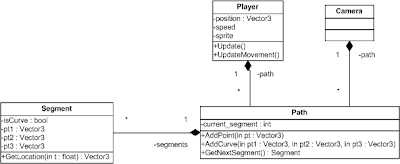
Here's a quick diagram I drew up after thinking about it a bit. I decided that it would be best to split the points into segments. Each segment would either contain 2 points if it was a linear path or 3 points if it was a bezier curve. A segment can return a specific point within it between 0 and 100% of the segment. A path is an ordered collection of segments. The player object will be able to query the Path for it's current segment, and then ask the segment its current location.
I think this will work out nicely, I have the header files laid out, I just need to write the implementation. One issue that may crop up is that the player and camera are going to have to communicate so the player can basically billboard so he's always facing the camera. Friending classes will probably work okay.
Lollerskivvies and I laid out a basic world design on the whiteboard today, I may visio-ify it later in the week. Basically, it got us really excited for the world design phase.. which should be coming up very soon!
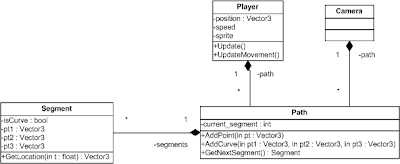
Here's a quick diagram I drew up after thinking about it a bit. I decided that it would be best to split the points into segments. Each segment would either contain 2 points if it was a linear path or 3 points if it was a bezier curve. A segment can return a specific point within it between 0 and 100% of the segment. A path is an ordered collection of segments. The player object will be able to query the Path for it's current segment, and then ask the segment its current location.
I think this will work out nicely, I have the header files laid out, I just need to write the implementation. One issue that may crop up is that the player and camera are going to have to communicate so the player can basically billboard so he's always facing the camera. Friending classes will probably work okay.
Lollerskivvies and I laid out a basic world design on the whiteboard today, I may visio-ify it later in the week. Basically, it got us really excited for the world design phase.. which should be coming up very soon!
Subscribe to:
Posts (Atom)